Using Docker with VS Code & ROS
Running on 18.04 or Windows, and need to build code in ROS-kinetic? Here’s how you do it.
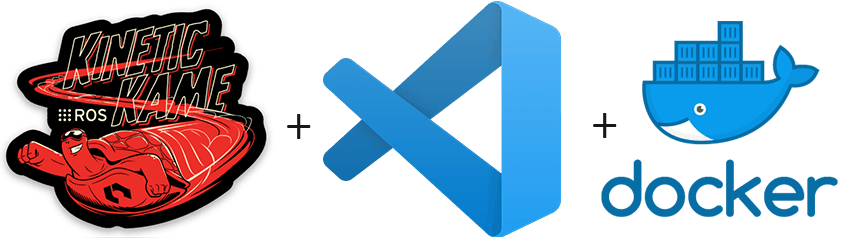
Acknowledgements
- Erdal from Erdal’s blog helped inspire this guide. I wanted to keep things a little more generic, and also use ROS-kinetic with catkin build tools for this example.
What You Need
Pre-reqs:
- Linux: Docker CE/EE 18.06+ and Docker Compose 1.21+. The snap version in Ubuntu 18.04 won’t do! Trust me, I’ve tried.
- Windows Docker Desktop 2.0+
Directory Structure
-
First make a directory structure somewhere on your computer like so. If you’re using windows, preferably don’t use your C: drive, but you can if you have to.
catkin_ws │ └───src | │___your_ros_pkgs | │ | │ | └───docker-setup.sh | | |____.devcontainer | |__devcontainer.json | |__Dockerfile
-
Don’t worry about what
docker-setup.sh, .devcontainer
are for now. Just create the.devcontainer, src
folders. Go ahead and drop whatever ROS Pkgs you have in src too.
VSCode
-
Go ahead and download the Remote Development Extension:
-
Create a
devcontainer.json
file in the .devcontainer folder that looks something like this:{ "name": "catkin-workspace", "dockerFile": "Dockerfile", "extensions": [ "ms-vscode.cpptools", "ms-iot.vscode-ros" ], "runArgs": [ "--cap-add=SYS_PTRACE", "--security-opt", "seccomp=unconfined", "-v", "${env:HOME}${env:USERPROFILE}/.ssh:/root/.ssh" ], "settings": { "terminal.integrated.shell.linux": "/bin/bash" }, "postCreateCommand": "bash /catkin_ws/src/docker-setup.sh", "workspaceMount": "src=path/to/catkin_ws/src/,dst=/catkin_ws/src/,type=bind,consistency=cached", "workspaceFolder": "/catkin_ws" }
Don’t forget to change “/path/to”!!
Docker
-
Create a
Dockerfile
also in .devcontainer that looks something like this:FROM ros:kinetic-ros-base RUN apt-get update && apt-get install -q -y \ python-catkin-tools python-pip RUN rm -rf /var/lib/apt/lists # good practice. RUN echo 'source /opt/ros/$ROS_DISTRO/setup.bash' >> /root/.bashrc
-
Now we also need to make sure we install the dependencies for your SRC folder. We will use a script that runs when setting up your container. Go ahead add a
docker-setup.sh
into the src folder with the following below:rosdep install -y -r --from-path /catkin_ws/
Ready to build the container!
-
Open up VS code, and open the catkin_ws folder. VS code should now detect the .devcontainer folder, and will ask you if you want to reopen this in a container. Go ahead and reopen it.
-
It’ll take a little bit to build. After it builds, go ahead and add a
.vscode
folder if there isn’t one already. Note that you must use the vscode app. -
Once you create the folder, go ahead and add these three files
settings.json, c_cpp_properties.json, tasks.json
://settings.json { "terminal.integrated.shellArgs.linux": [ "-l", "-i" ], "ros.distro": "kinetic", "python.linting.enabled": true, "python.autoComplete.extraPaths": [ "/golfcart_ws/devel/lib/python2.7/dist-packages", "/opt/ros/kinetic/lib/python2.7/dist-packages" ], "python.linting.pylintArgs": [ "--init-hook"] }
//c_cpp_properties.json { "configurations": [ { "browse": { "databaseFilename": "", "limitSymbolsToIncludedHeaders": true }, "includePath": [ "/golfcart_ws/devel/include/**", "/opt/ros/kinetic/include/**", "/usr/include/**" ], "name": "ROS", "intelliSenseMode": "gcc-x64", "compilerPath": "/usr/bin/gcc", "cStandard": "c11", "cppStandard": "c++11" } ], "version": 4 }
//tasks.json { // See https://go.microsoft.com/fwlink/?LinkId=733558 // for the documentation about the tasks.json format "version": "2.0.0", "tasks": [ { "label": "catkin build", "type": "shell", "command": "catkin build --cmake-args -DCMAKE_BUILD_TYPE=Release -DCMAKE_EXPORT_COMPILE_COMMANDS=ON -DCMAKE_CXX_STANDARD=11", "problemMatcher": [], "group": { "kind": "build", "isDefault": true } }, { "label": "catkin run_tests", "type": "shell", "command": "catkin run_tests", "problemMatcher": [], "group": { "kind": "test", "isDefault": true } }, ] }
-
Now all you gotta do is build it! Press
Crtl + Shift + B
and watch the magic happen!